IPConnect class¶
IPConnect
provides the means of connecting ports and interfaces of objects that are subclasses of Wrapper
.
Since IPConnect
is a subclass of Wrapper
itself, this means that it also has IO - ports and interfaces, and that multiple IPConnect
s can have their ports and interfaces connected to each other (or other objects that subclass Wrapper
).
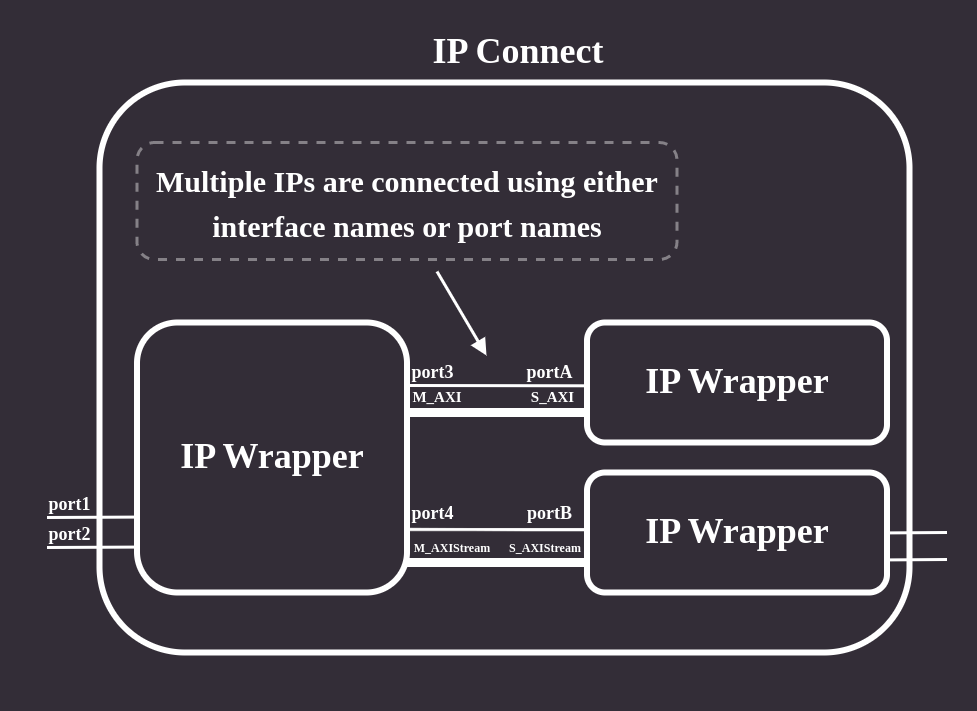
Instances of Wrapper
objects can be added to an IPConnect
using add_component()
method:
# create an IP wrapper
dma = IPWrapper('DMATop.yaml', ip_name='DMATop', instance_name='DMATop0')
ipc = IPConnect()
ipc.add_component("dma", dma)
Connections between cores can then be made with connect_ports()
and connect_interfaces()
based on names of the components and names of ports/interfaces:
ipc.connect_ports("comp1_port_name", "comp1_name", "comp2_port_name", "comp2_name")
ipc.connect_interfaces("comp1_interface_name", "comp1_name", "comp2_interface_name", "comp2_name")
Setting ports or interfaces of a module added to IPConnect
as external with _set_port()
and _set_interface()
and allows these ports/interfaces to be connected to other Wrapper
instances.
ipc._set_port("comp1_name", "comp1_port_name", "external_port_name")
ipc._set_interface("comp1_name", "comp1_interface_name", "external_interface_name")
This is done automatically in the make_connections()
method when the design is built, based on the data from the YAML design description.
- class IPConnect(*args, src_loc_at=0, **kwargs)¶
Connector for multiple IPs, capable of connecting their interfaces as well as individual ports.
- __init__(name: str = 'ip_connector')¶
- _connect_external_ports(internal: WrapperPort, external: WrapperPort)¶
Makes a pass-through connection - port of an internal module in IPConnect is connected to an external IPConnect port.
- Parameters:¶
- internal: WrapperPort¶
port of an internal module of IPConnect
- external: WrapperPort¶
external IPConnect port
- _connect_internal_ports(port1: WrapperPort, port2: WrapperPort)¶
Connects two ports with matching directionality. Disallowed configurations are: - input to input - output to output - inout to inout All other configurations are allowed.
- Parameters:¶
- port1: WrapperPort¶
1st port to connect
- port2: WrapperPort¶
2nd port to connect
- _connect_to_external_port(internal_port: str, internal_comp: str, external_port: str, external: DesignExternalPorts) None ¶
Connect internal port of a component to an external port
- Parameters:¶
- internal_port: str¶
internal port name in internal_component to connect to external_port
- internal_comp: str¶
internal component name
- external_port: str¶
external port name
- external: DesignExternalPorts¶
dictionary in the form of {“in”: list, “out”: list, “inout”: list} containing port names specified as external in each of the three categories. All keys are optional and lack of a category implies an empty list
- _set_interface(comp_name: str, iface_name: str, external_iface_name: str) None ¶
Set interface specified by name as an external interface
- _set_port(comp_name: str, port_name: str, external_name: str) None ¶
Set port specified by name as an external port
- add_component(name: str, component: Wrapper) None ¶
Add a new component to this IPConnect, allowing to make connections with it
- connect_interfaces(iface1: str, comp1_name: str, iface2: str, comp2_name: str) None ¶
Make connections between all matching ports of the interfaces
- connect_ports(port1_name: str, comp1_name: str, port2_name: str, comp2_name: str) None ¶
Connect ports of IPs previously added to this Connector
- get_ports() list ¶
Return a list of external ports of this module
- make_connections(ports: DS_PortsT, interfaces: DS_InterfacesT, external: DesignExternalSection) None ¶
Use names of port and names of ips to make connections
- make_interconnect_connections(interconnects: Dict[str, DesignSectionInterconnect], external: DesignExternalSection)¶
Connect subordinates and managers to their respective interfaces in the interconnect